The Import API can be used to create or update a number of entities in bulk. This includes branches (companies), users, bookable staff (people) and their schedules.
Payload
{
meta: {
feed_name: "My import"
},
settings: {},
data: {
companies: [<Company>],
people: [<Person>],
users: [<User>]
}
}
"data": {
"companies": [
{
"external_id": "bank_branch1",
"object_type": "company",
"name": "Bank Branch 1",
"created_at": "{{env_yesterday}}",
"last_modified": "2023-09-15T08:35:38.218Z",
"status": "live",
"telephone": "+61 8 8203 7973",
"parent_external_id": "bank_parent",
"base_company_ref": "bank_parent",
"member_company_ref": "bank_parent",
"address": {
"address1": "re Terrace",
"address4": "NORTH ADELAIDE",
"address5": "SA",
"postcode": "5006",
"country": "Australia"
},
"timezone": "Australia/Adelaide",
"opening_hours": {
"days": [
{
"day": "Monday",
"start": "10:00",
"end": "19:00"
},
{
"day": "Tuesday",
"start": "10:00",
"end": "19:00"
},
{
"day": "Wednesday",
"start": "10:00",
"end": "19:00"
},
{
"day": "Thursday",
"start": "10:00",
"end": "19:00"
},
{
"day": "Friday",
"start": "10:00",
"end": "19:00"
},
{
"day": "Saturday",
"start": "10:00",
"end": "19:00"
},
{
"day": "Sunday",
"start": "12:00",
"end": "18:00"
}
]
}
}
],
"people": [
{
"external_id": "teller_1",
"object_type": "person",
"name": "Teller 1",
"email": "[email protected]",
"telephone": "08 9999-1234",
"status": "enabled",
"created_at": "{{env_yesterday}}",
"last_modified": "2023-09-15T08:35:38.218Z",
"bookables": [
{
"company_external_id": "bank_branch1",
"assignments": [
{
"service_external_id": "mortgage_advice"
},
{
"service_external_id": "open_an_account"
}
]
}
],
"company_external_ids": [
"bank_branch1"
],
"schedules": {
"schedules_type": "shifts",
"shifts": [
{
"when": "2023-03-01",
"where": [
"bank_branch1"
],
"shift": [
{
"start_time": "11:00",
"end_time": "17:00"
}
]
},
{
"when": "2023-03-02",
"where": [
"bank_branch1"
],
"shift": [
{
"start_time": "09:00",
"end_time": "18:00"
}
]
}
]
}
},
{
"external_id": "teller_2",
"object_type": "person",
"name": "Teller 2",
"email": "[email protected]",
"telephone": "08 9999-1234",
"status": "enabled",
"created_at": "{{env_yesterday}}",
"last_modified": "2023-09-15T08:35:38.218Z",
"bookables": [
{
"company_external_id": "bank_branch2",
"assignments": [
{
"service_external_id": "mortgage_advice"
},
{
"service_external_id": "open_an_account"
}
]
}
],
"company_external_ids": [
"bank_branch2"
],
"schedules": {
"schedules_type": "shifts",
"shifts": [
{
"when": "2023-03-01",
"where": [
"bank_branch2"
],
"shift": [
{
"start_time": "11:00",
"end_time": "17:00"
}
]
},
{
"when": "2023-03-02",
"where": [
"bank_branch2"
],
"shift": [
{
"start_time": "09:00",
"end_time": "18:00"
}
]
}
]
}
}
],
"users": [
{
"company_external_ids": [
"bank_parent"
],
"name": "Teller 1",
"email": "[email protected]",
"roles": [
"user"
],
"status": "live",
"external_id": "teller_1",
"object_type": "user",
"created_at": "{{env_yesterday}}",
"last_modified": "2023-09-15T08:35:38.218Z"
}
]
}
}
Company
The hierarchy of an organisation can be represented using company objects. Companies can represent individual branches (where appointments, events or queuing services take place), departments or regions.
Settings associated with companies drive the available options throughout the system, such as whether events or services are available, if prices are used and what languages are available for translating the customer and / or Studio administration journey user interfaces.
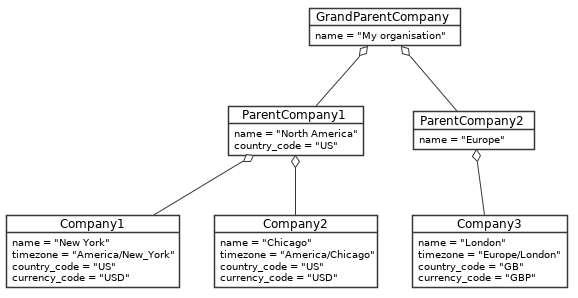
Sample Company only payload
The Import API allows you to send separate payloads for each of the objects. Below is a sample locations payload demonstrating the creation of a Parent and Child location.
{
"meta": {
"version": "0.0.1",
"created_at": "{{now}}",
"callback_url": "http://example.com/something.system",
"feed_name": "customer_import_{{now}}"
},
"settings": {
"actions": [
"create",
"update",
"activate"
]
},
"data": {
"companies": [
{
"external_id": "physical_parent",
"object_type": "company",
"name": "Physical Parent",
"company_type": "physical",
"status": "live",
"created_at": "{{env_now}}",
"last_modified": "{{env_now}}",
"is_parent": true,
"parent_external_id": "GrandParent",
"base_company_ref": "physical_parent",
"member_company_ref": "physical_parent",
"address": {
"line_1": " ",
"line_2": null,
"locality": null,
"region": null,
"postal_code": null,
"country": "United Kingdom"
},
"currency_code": "GBP",
"timezone": "Europe/London",
"country_code": "gb"
"resources": [
{
"description": "The one with beautiful windows",
"name": "Video Conference",
"telephone": "0207 432 5439",
"external_id": "436b:6258:81fa:b1b4:284c:99e8:8fe9:19b",
"object_type": "resource",
"status": "live",
"created_at": "2018-11-11T15:31:49Z",
"last_modified": "2018-11-27T15:31:49Z"
},
{
"description": "The one with the whiteboard",
"name": "Meeting Room A",
"telephone": "0207 432 5439",
"external_id": "6ee2:afc3:2f91:cf8c:4d3f:14e:6ea8:1cfe",
"object_type": "resource",
"status": "live",
"created_at": "2018-11-15T15:31:49Z",
"last_modified": "2018-11-22T15:31:49Z"
}
]
},
{
"external_id": "physical_store_1",
"object_type": "company",
"name": "Physical Store for Appointments",
"company_type": "physical",
"status": "live",
"telephone": null,
"created_at": "{{env_yesterday}}",
"last_modified": "{{env_now}}",
"is_parent": false,
"parent_external_id": "physical_parent",
"base_company_ref": "physical_parent",
"member_company_ref": "physical_parent",
"address": {
"line_1": "10 Downing Street ",
"line_2": null,
"locality": null,
"region": "LONDON",
"postal_code": "EC1A 1BB",
"country": "United Kingdom"
},
"currency_code": "GBP",
"timezone": "Europe/London",
"country_code": "gb",
"opening_hours": {
"days": [
{
"day": "Tuesday",
"start": "09:30",
"end": "16:30"
},
{
"day": "Wednesday",
"start": "09:30",
"end": "16:30"
},
{
"day": "Thursday",
"start": "09:30",
"end": "16:30"
},
{
"day": "Friday",
"start": "09:30",
"end": "16:30"
},
{
"day": "Saturday",
"start": "09:30",
"end": "13:30"
]
}
}
]
}
}
Property name | Required on creation | Type | Description |
---|---|---|---|
object_type | True | String | "company" |
name | True | String | Name of branch / location |
description | False | String | Description of branch |
address.line1 | True | String | Address line 1 |
address.locality | False | String | Address locality |
address.state | False | String | Address state |
address.postal_code | False | String | Address postal code |
address.country | True | String | Address Country e.g. United States, United Kingdom, France, Germany |
timezone | True | String | Time zone e.g. CET - ‘Europe/Paris’ GMT - ‘Europe/London’ EST- ‘America/New_York’ CST- ‘America/Chicago’ MST- ‘America/Denver’ PST- ‘America/Los_Angeles’ AKST - ‘America/Anchorage’ HST- ‘Pacific/Honolulu’ |
status | False | String | Whether the location is Disabled. There are 3 possible values. live, disabled, delete. |
created_at | False | Datetime | This will be automatically set if not provided |
external_id | True | String | Used to find the object model within JRNI. This is a primary key field. Adding the same records with a different key will result in duplicate records. |
last_modified | True | Datetime | Used to determine if an object should be updated |
is_parent | True | Boolean | Used to create a Parent company to house child bookable branches / locations. Set to false when creating child locations. Set to true when creating a Parent company |
parent_external_id | True | String | Used to associate a child location to a specific parent company where there is a Grandparent / Parent / Child company configuration. Set the external id of the Grandparent company when creating a Parent company. Set to the Parent company when creating a child location under a specific parent. |
base_company_ref | False | String | |
member_company_ref | False | String | Sets where to store end customer data in JRNI |
User
Your internal staff who will administer data in the JRNI platform, manage the bookable staff within the JRNI platform and / or staff who make or take bookings on behalf of customers, eg Call Centre / Counter staff making bookings. Essentially a user login to the JRNI platform.
Refer to JRNI User roles and permissions
Property name | Required on creation | Type | Description |
---|---|---|---|
object_type | True | String | "user" |
name | True | String | Name associated with user |
True | String | Email associated with user | |
roles | True | Array[String] | A role associated with the user. The possible values are: admin, user, and owner. |
company_external_ids | True | Array[String] | A list of companies the user has access to. |
created_at | False | Datetime | This will be automatically set if not provided. |
external_id | True | String | Used to find the object model within JRNI. This is a primary key field. Adding the same records with a different key will result in duplicate records. |
last_modified | True | Datetime | Used to decide if an object should be updated. |
status | True | String | There are 3 possible values. live, disabled, delete. |
person_external_id | False | String | ID of associated bookable person |
Person
A person in JRNI is a bookable staff member who conducts appointments, hosts events or serves customers in a queue.
Refer to:-JRNI - Creating staff members
Sample Staff only payload with examples of Schedules upload
{
"meta": {
"version": "0.0.1",
"created_at": "{{env_now}}",
"callback_url": "http://example.com/something.system",
"feed_name": "example_staff_only_import_{{env_now}}"
},
"settings": {
"actions": [
"create",
"update",
"activate"
]
},
"data": {
"people": [
{
"external_id": "shop4genstaff",
"object_type": "person",
"name": "Unassigned - Shop4",
"status": "live",
"group": "Online Bookings",
"never_booked": true,
"created_at": "{{env_yesterday}}",
"last_modified": "{{env_now}}",
"bookables": [
{
"company_external_id": "Shop4",
"assignments": [
{
"service_external_id": "MMU"
},
{
"service_external_id": "personal_shopper"
}
]
}
],
"company_external_ids": [
"Shop4"
],
"schedules": {
"schedules_type": "custom",
"shift_patterns": [
{
"company_external_id": [
"Shop4"
],
"start_date": "2023-01-01",
"end_date": "2030-12-31",
"start_time": "10:00",
"end_time": "12:00",
"frequency": "weekly",
"interval": 1,
"by_day": [
"Monday"
],
"except_date": [
"2024-12-25",
"2025-12-25",
"2026-12-25",
"2027-12-25",
"2028-12-25",
"2029-12-25",
"2030-12-25"
],
"shift_pattern_condition_id": "default"
},
{
"company_external_id": [
"Shop4"
],
"start_date": "2023-01-01",
"end_date": "2030-12-31",
"start_time": "13:00",
"end_time": "17:00",
"frequency": "weekly",
"interval": 2,
"by_day": [
"Tuesday","Wednesday","Thursday"
],
"except_date": [
"2024-12-25",
"2025-12-25",
"2026-12-25",
"2027-12-25",
"2028-12-25",
"2029-12-25",
"2030-12-25"
],
"shift_pattern_condition_id": "default"
}
]
}
},
{
"external_id": "dmimieux",
"object_type": "person",
"name": "Dan Mimieux",
"email": "[email protected]",
"telephone": "44 99999-1234",
"status": "disabled",
"group": null,
"created_at": "{{env_yesterday}}",
"last_modified": "{{env_now}}",
"bookables": [
{
"company_external_id": "Shop4",
"assignments": [
{
"service_external_id": "MMU"
},
{
"service_external_id": "personal_shopper"
}
]
}
],
"company_external_ids": [
"Shop4"
],
"schedules": {
"schedules_type": "shifts",
"shifts": [
{
"when": "2023-05-01",
"where": [
"Shop4"
],
"shift": [
{
"start_time": "11:00",
"end_time": "17:00"
}
]
},
{
"when": "2023-05-02",
"where": [
"Shop4"
],
"shift": [
{
"start_time": "09:00",
"end_time": "18:00"
}
]
},
{
"when": "2023-05-06",
"where": [
"Shop4"
],
"shift": [
{
"start_time": "09:00",
"end_time": "18:00"
}
]
},
{
"when": "2023-05-07",
"where": [
"Shop4"
],
"shift": [
{
"start_time": "09:00",
"end_time": "18:00"
}
]
},
{
"when": "2023-05-10",
"where": [
"Shop4"
],
"shift": [
{
"start_time": "09:00",
"end_time": "18:00"
}
]
},
{
"when": "2023-05-11",
"where": [
"Shop4"
],
"shift": [
{
"start_time": "09:00",
"end_time": "15:00"
}
]
}
]
}
}
]
}
}
Property name | Required on creation | Type | Description | Visible in journey |
---|---|---|---|---|
object_type | True | String | "person" | No |
name | True | String | Used to display name of person | Yes |
description | False | String | A description of the person | No |
created_at | False | Datetime | This will be automatically set if not provided | No |
company_external_ids | False | Array[String] | A list of companies related to the person | No |
group | False | String | Assign the person to a staff group. | Yes |
False | String | An email associated with the person | No | |
telephone | False | String | A phone number associated with the person | No |
extras | False | Array[Extra] | See Extra | No |
schedule | False | Array[Schedule] | See Schedule | Determines when a person can take appointments |
last_modified | True | Datetime | Used to determine whether an object should be updated | No |
status | True | String | There are 3 possible values. live, disabled, deleted | No |
ews | False | Object | Email address for an Exchange Integration mailbox e.g. "ews": { "email": "[email protected]", "enabled": true }, | |
eoc | False | Object | Email address for an Exchange Online Integration mailbox e.g. "eoc": { "email": "[email protected]", "enabled": true }, | |
bookables | False | Array[Bookable] | See Bookable | |
group | False | String | Name of group the person belongs to. If it does not already exist, it will be created. |
Bookable
Determines which services the person can deliver at a particular location.
"bookables": [
{
"company_external_id": "bank_branch1",
"assignments": [
{
"service_external_id": "mortgage_advice"
},
{
"service_external_id": "open_an_account"
}
]
}
Property name | Required on creation | Type | Description |
---|---|---|---|
company_external_id | True | String | The external reference for the location the assignments apply to |
assignments | True | Array[Service] | See Service |
Service
Property name | Required on creation | Type | Description |
---|---|---|---|
service_external_id | True | String | The service external reference |
Schedule
Indicates the periods of time when the person will be available to be booked.
Property name | Required on creation | Type | Description |
---|---|---|---|
schedule_type | False | String | The format used for the schedule. Can be default, custom or shift. |
shifts | False | Array[Shift] | Only include if using the shift schedule type |
shift_patterns | False | Array[ShiftPattern] | Only include if using the shift_pattern schedule type |
ShiftPattern
A schedule can be formed with 1 or many shift patterns to allow for varying staff work rotas. A standard shift pattern could be Monday to Friday 09:00 to 17:00 and repeats weekly.
Sample shift pattern payload array
"schedules": {
"schedules_type": "custom",
"shift_patterns": [
{
"company_external_id": [
"Shop4"
],
"start_date": "2023-01-01",
"end_date": "2030-12-31",
"start_time": "10:00",
"end_time": "12:00",
"frequency": "weekly",
"interval": 1,
"by_day": [
"Monday"
],
"except_date": [
"2024-12-25",
"2025-12-25",
"2026-12-25",
"2027-12-25",
"2028-12-25",
"2029-12-25",
"2030-12-25"
],
"shift_pattern_condition_id": "default"
},
Property name | Required on creation | Type | Description |
---|---|---|---|
frequency | True | String | The allowed values are: weekly, daily |
start_date | True | String | Format: YYYY-MM-DD |
start_time | True | String | Format: HH:mm The hours must have two digits. For example, use “09:00”. |
end_time | True | String | Format: HH:mm The hours must have two digits. For example, use “09:00”. |
by_day | True | Array[String] | Possible values: monday, tuesday, wednesday, thursday, friday, saturday and sunday. |
except_date | False | Array[String] | Format: YYYY-MM-DD |
company_external_ids | True | Array[String] | Represents the locations to which the schedule applies. The locations are identified using the external_id |
methods_of_appointment | False | Array[String] | Possible values: In-person, Phone, Video |
Shift
A shift is a individual or specific date of availability
Sample Schedule using Shifts
"schedules": {
"schedules_type": "shifts",
"shifts": [
{
"when": "2023-03-01",
"where": [
"bank_branch2"
],
"shift": [
{
"start_time": "11:00",
"end_time": "17:00"
}
]
},
{
"when": "2023-03-02",
"where": [
"bank_branch2"
],
"shift": [
{
"start_time": "09:00",
"end_time": "18:00"
}
]
}
]
Property name | Required on creation | Type | Description |
---|---|---|---|
when | True | String | Format: YYYY-MM-DD |
where | True | Array[String] | Represents the locations to which the schedule applies. The locations are identified using the external_id |
how | False | Array[String] | Possible values: In-person, Phone, Video |
shifts | True | Array[ShiftTimes] |
ShiftTimes
Property name | Required on creation | Type | Description |
---|---|---|---|
start_time | True | String | Format: HH:mm The hours must have two digits. For example, use “09:00”. |
end_time | True | String | Format: HH:mm The hours must have two digits. For example, use “09:00”. |
Errors
Object | Status | Error message | Cause/Resolution |
---|---|---|---|
Company | Failed | last_modified must be in ISO-8601 format | |
Company | Skipped | Unable to create due to missing action 'create' | |
Company | Skipped | Unable to update due to missing action 'update' | |
Company | Skipped | last_modified: this object is older than the one in BookingBug | The updated date in JRNI is newer than that last modified date in the import payload. Update the last modified date in the import file to the current date and time. |
Company | Failed | object to import is missing required key: name | |
Company | Failed | object to import is missing required key: status | |
Company | Failed | object to import is missing required key: object_type | |
Company | Failed | object to import has object_type 'x' but we were expecting 'company' | |
Company | Failed | parent_external_id was specified but the company could not be found | |
Company | Failed | base_company_ref was specified but the company could not be found | |
Company | Failed | member_company_ref was specified but the company could not be found | |
Company | Failed | invalid currency code supplied for company | |
Company | Failed | invalid name, cannot be blank | |
Person | Failed | last_modified must be in ISO-8601 format | |
Person | Skipped | Unable to create due to missing action 'create' | |
Person | Skipped | Unable to update due to missing action 'update' | |
Person | Skipped | last_modified: this object is older than the one in BookingBug | The updated date in JRNI is newer than that last modified date in the import payload. Update the last modified date in the import file to the current date and time. |
Person | Failed | object to import is missing required key: object_type | |
Person | Failed | object to import has object_type 'x' but we were expecting 'person' | |
Person | Failed | company to add person to does not exist. | Check the company has imported into JRNI and is not disabled or deleted. Check that a company with that external ID was included in the company section of the import file and successfully imported. |
Person | Failed | Unrecognised 'schedules_type'. We only currently support 'default'; 'shifts' or 'custom'. | |
Person | Failed | Email is not a valid email address | Check email address is valid |
Person | Failed | Failed to create object mapping for <email_address>. The requested user is invalid. | Failed to create calendar connection due to invalid email account in customer side calendar configuration |
Person | Failed | Failed to create object mapping for <email_address>. User with email address <email_address> already exists. | Calendar connection already exists in JRNI for this staff member email account. |
Schedule | Failed | Invalid date: . Date format is 'YYYY-MM-DD' | |
Schedule | Failed | Malformed: by_day | Ensure the values for by_day are in the list monday, tuesday, wednesday, thursday, friday |
Schedule | Failed | Missing: by_day | If the shift pattern is recurring weekly, by_day must be included. |
Schedule | Failed | Malformed times: start_time(<start_time>) is passed or equal the end_time(<end_time>) | Check the start and end times for the shift. |
Schedule | Failed | Malformed or missing: start_date | |
Schedule | Failed | Malformed or missing: frequency | |
Schedule | Failed | Malformed methods_of_appointment: <bad_value> | Ensure the values for methods_of_appointment are in the list In-person, Phone, Video |
Schedule | Failed | The shift pattern is colliding with the . The overlap is between <start_time> and <end_time> on the | Ensure the shifts are not overlapping |
User | Failed | last_modified must be in ISO-8601 format | |
User | Skipped | Unable to create due to missing action 'create' | |
User | Skipped | Unable to update due to missing action 'update' | |
User | Skipped | last_modified: this object is older than the one in BookingBug | The updated date in JRNI is newer than that last modified date in the import payload. Update the last modified date in the import file to the current date and time. |
User | Failed | object to import is missing required key: name | |
User | Failed | object to import is missing required key: email | |
User | Failed | object to import is missing required key: roles | |
User | Failed | object to import is missing required key: object_type | |
User | Failed | object to import has object_type 'x' but we were expecting 'user' | |
User | Failed | No companies to assign the user to | |
User | Failed | One of the companies has not been imported yet | |
User | Failed | E-mail already in use by another user | |
User | Failed | Invalid e-mail | |
User | Failed | Invalid name | |
User | Failed | User objects can currently only have 1 role. The Array is for future proofing | |
User | Failed | Invalid roles | |
User | Failed | Could not find associated person object for user |